Essential JavaScript Array Methods
- With Code Example
- February 28, 2024
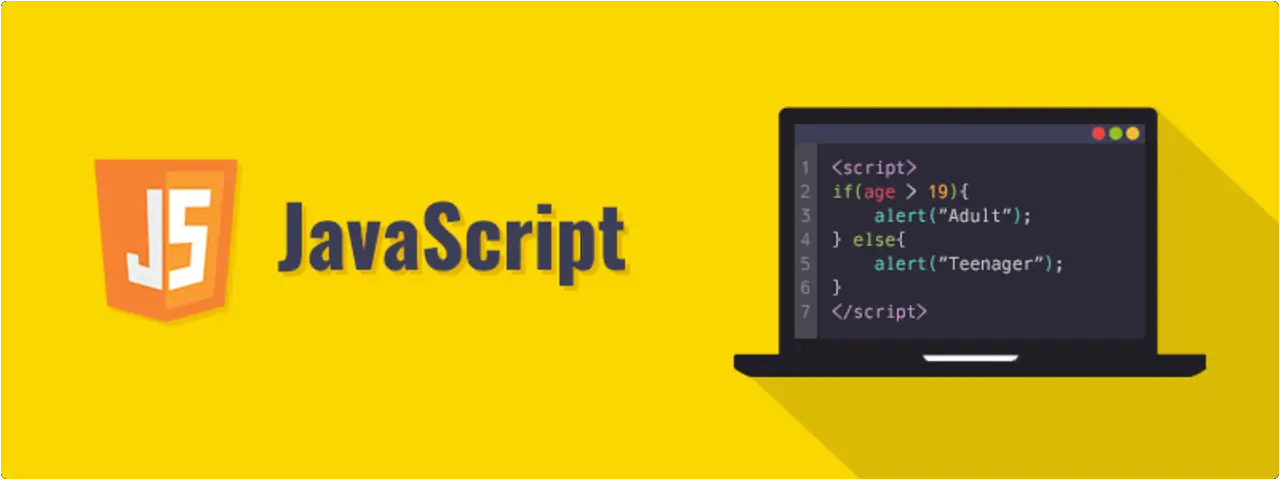
JavaScript arrays are like supreme storehouses that help us organize and bundle multiple values together. The purpose of this article is to walk you through the array manipulation domain. We will be studying some core and widely applied JavaScript array methods, which can considerably simplify your work as a programmer who deals with such mighty data structures.
Introduction
JavaScript arrays play a pivotal role in modern web development, offering a structured approach to organizing and managing collections of data. With the need for efficient manipulation of arrays becoming increasingly prevalent in web applications, understanding built-in array methods is paramount. These methods streamline array manipulation tasks, enhance code readability, and empower developers to build robust and dynamic applications.
Essential Methods
1. forEach():
forEach
allows us to iterate over each element in an array and execute a provided callback function.
const numbers = [1, 2, 3];
numbers.forEach(num => console.log(num * 2));
Output:
2
4
6
2. map
:
map
creates a new array by applying a callback function to each element of the original array.
const numbers = [1, 2, 3];
const squares = numbers.map(num => num * num);
console.log(squares); // [1, 4, 9]
3. filter
:
filter
generates a new array containing elements that pass a specified test implemented in a callback function.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
console.log(evenNumbers); // [2, 4]
4. reduce
:
reduce` applies a callback function against an accumulator and each element in the array to reduce it to a single value.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0);
console.log(sum); // 15
Additional Methods
In addition to the essential methods discussed above, JavaScript provides several other powerful array methods:
1. push()
and pop()
:
Adding and Removing Elements from the End of an Array
The push()
method adds one or more elements to the end of an array, while the pop()
method removes the last element from an array and returns that element.
// Using push()
const fruits = ['apple', 'banana', 'orange'];
fruits.push('grape', 'kiwi');
console.log(fruits); // Output: ['apple', 'banana', 'orange', 'grape', 'kiwi']
// Using pop()
const removedFruit = fruits.pop();
console.log(removedFruit); // Output: 'kiwi'
console.log(fruits); // Output: ['apple', 'banana', 'orange', 'grape']
2. unshift()
and shift()
:
Adding and Removing Elements from the Beginning of an Array
The unshift()
method adds one or more elements to the beginning of an array, while the shift()
method removes the first element from an array and returns that element.
// Using unshift()
const colors = ['red', 'blue', 'green'];
colors.unshift('yellow', 'orange');
console.log(colors); // Output: ['yellow', 'orange', 'red', 'blue', 'green']
// Using shift()
const removedColor = colors.shift();
console.log(removedColor); // Output: 'yellow'
console.log(colors); // Output: ['orange', 'red', 'blue', 'green']
3. indexOf()
:
Finding the Index of a Specified Item in an Array
The indexOf()
method returns the first index at which a given element can be found in the array, or -1 if it is not present.
const numbers = [1, 2, 3, 4, 5];
const index = numbers.indexOf(3);
console.log(index); // Output: 2 (index of element 3 in the array)
4. slice()
:
Extracting a Portion of an Array into a New Array
The slice()
method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) where start and end represent the index of items in that array.
const fruits = ['apple', 'banana', 'orange', 'grape', 'kiwi'];
const slicedFruits = fruits.slice(1, 4);
console.log(slicedFruits); // Output: ['banana', 'orange', 'grape']
5. sort()
:
Sorting Array Elements Alphabetically or Numerically
The sort()
method sorts the elements of an array in place and returns the sorted array. By default, the elements are sorted as strings in alphabetical and ascending order.
const fruits = ['banana', 'apple', 'orange', 'grape', 'kiwi'];
fruits.sort();
console.log(fruits); // Output: ['apple', 'banana', 'grape', 'kiwi', 'orange']
6. find()
:
Finding the First Element in an Array that Satisfies a Specified Condition
The find()
method returns the first element in the array that satisfies the provided testing function. Otherwise, it returns undefined
.
const numbers = [10, 20, 30, 40, 50];
const foundNumber = numbers.find(number => number > 25);
console.log(foundNumber); // Output: 30
7. lastIndexOf()
:
Returns the last index at which a given element can be found in the array, or -1 if it is not present.
const numbers = [2, 5, 9, 2];
console.log(numbers.lastIndexOf(2)); // Output: 3
console.log(numbers.lastIndexOf(7)); // Output: -1 (not found)
console.log(numbers.lastIndexOf(2, 2)); // Output: 0
console.log(numbers.lastIndexOf(2, -2)); // Output: 0
console.log(numbers.lastIndexOf(2, -1)); // Output: 3
Choosing the Right Method
When selecting an array method for a specific task, it’s essential to consider factors such as performance, readability, and the desired outcome. Understanding the nuances and capabilities of each method enables developers to make informed decisions and write efficient, maintainable code.
Practical Applications
The versatility of JavaScript array methods extends to a wide range of real-world applications:
- Data Filtering and Transformation: Preparing data for display or analysis by filtering and transforming arrays based on specific criteria.
- Form Data Handling: Processing user input from web forms, validating inputs, and formatting responses using array manipulation techniques.
- Statistical Calculations: Analyzing numerical datasets to compute averages, sums, or identify outliers using array aggregation methods.
Conclusion
In conclusion, mastering JavaScript array methods empowers developers to efficiently manipulate data structures, streamline code, and build robust applications. By leveraging the diverse functionalities of array methods, developers can tackle complex array manipulation tasks with confidence and precision. Whether you’re filtering, transforming, or aggregating array data, JavaScript array methods offer a powerful arsenal of tools to meet your programming needs. Embrace the versatility of array methods and unlock new possibilities in your JavaScript projects. Happy coding!