Exploring The ForEach Loop In JavaScript
- With Code Example
- February 27, 2024
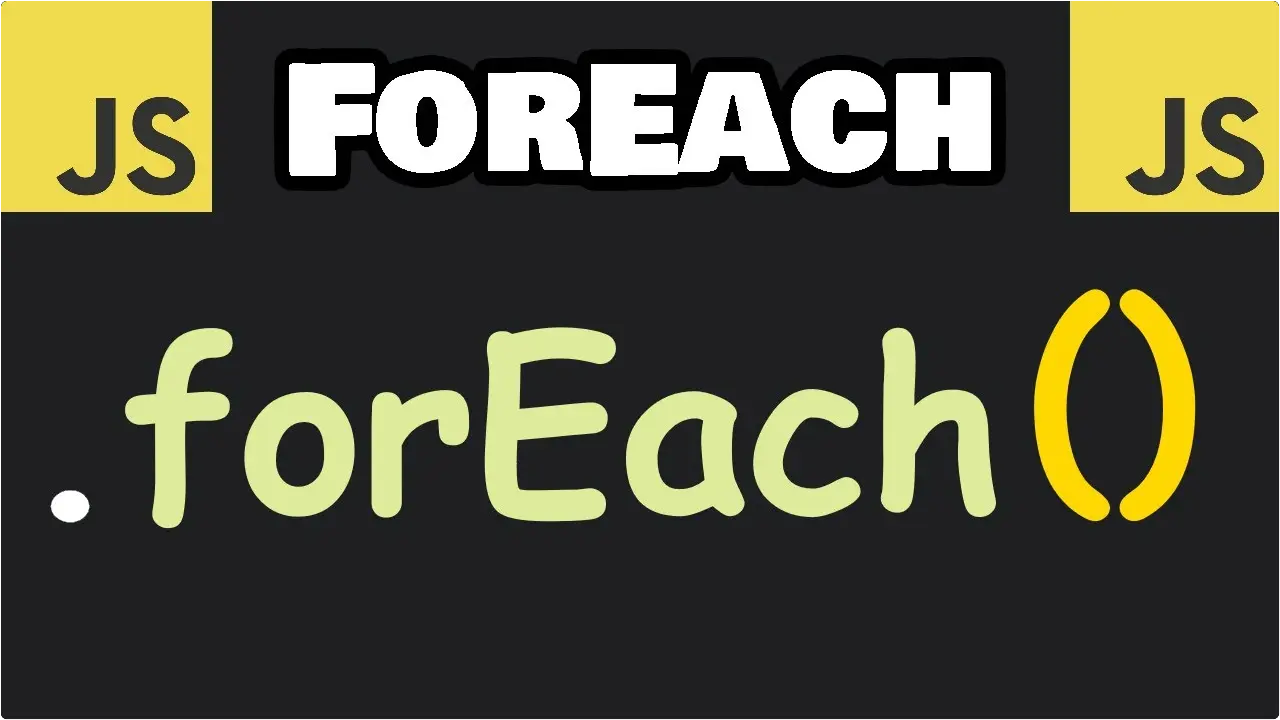
Working with arrays is such an important part of writing JavaScript. We’re always looping
through them to filter data, transform it, or process it in some way. But all that looping can get messy pretty fast. Luckily, JavaScript arrays give us a great tool to handle it cleanly - the forEach
method.
In this guide, we’ll dive into how to use forEach
. I’ll walk through the syntax, parameters, and some practical examples so you can see it in action. We’ll also cover key points to remember and some advanced tips and tricks for levelling up your forEach
skills.
Table of Contents
By the end, you’ll have a solid understanding of how to use this powerful method to loop through arrays like a pro. Your JavaScript
code will be cleaner, shorter, and more efficient. Iteration is a core array manipulation skill, and forEach
helps us handle it with ease. So let’s get looping!
You Will Learn
- π‘ Working with arrays in JavaScript involves looping through them to filter, transform, or process data
- π‘ The forEach method in JavaScript arrays allows for clean iteration over elements
- π‘ Syntax of forEach: array.forEach(callbackFunction)
- π‘ Practical examples of forEach: printing elements, modifying array elements, performing calculations
- π‘ Key points and considerations: forEach does not return a new array, has limitations compared to other array methods
- π‘ Comparison with other array methods like map, filter, and reduce is important for choosing the right tool
- π‘ Conclusion: forEach is a valuable tool for array manipulation in JavaScript
- π‘ Advanced tips include using arrow functions for readability and considering alternatives like map, filter, and reduce.
Understanding forEach
The forEach
method is an integral part of the JavaScript language, specifically implemented as a member of the Array
prototype. Introduced in ECMAScript 5 (ES5), it has become a staple feature of modern JavaScript development, widely supported across major browsers and JavaScript environments. Unlike traditional iterative approaches using for
loops or the newer for...of
loops, forEach
offers a more declarative and expressive syntax for iterating over arrays, promoting code readability and maintainability.
Syntax and Parameters
Let’s break down the syntax of forEach
:
array.forEach(callbackFunction)
Here, array
represents the array on which the forEach
method is called, and callbackFunction
is the function provided to forEach
as an argument. The callbackFunction
is invoked once for each element in the array, receiving three arguments:
element
: The current element being processed during iteration.index
: The index of the current element within the array.array
: The array on whichforEach
is applied.
Additionally, forEach
can accept an optional fourth parameter thisArg
, which specifies the value to be used as this
within the callbackFunction
, allowing for explicit context binding if needed.
Practical Examples
Let’s dive into practical examples to illustrate the versatility of forEach
:
1. Printing each element of an array:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach(element => console.log(element));
Here, the forEach
loop iterates over each element in the numbers
array, logging each element to the console.
2. Modifying elements within the array:
const names = ['Alice', 'Bob', 'Charlie'];
names.forEach((name, index, array) => array[index] = name.toUpperCase());
In this example, forEach
is used to iterate over the names
array, converting each name to uppercase and updating the original array in place.
3. Performing calculations based on array elements:
const prices = [10, 20, 30];
let totalPrice = 0;
prices.forEach(price => totalPrice += price);
Here, forEach
is employed to iterate over the prices
array, accumulating the total price by summing up all the individual prices.
4. Accessing index and original array within the callback:
const fruits = ['Apple', 'Banana', 'Orange'];
fruits.forEach((fruit, index, array) => console.log(`${fruit} is at index ${index} of ${array}`));
In this example, the forEach
loop provides access to the index and the original array, facilitating more dynamic processing of array elements.
Key Points and Considerations
While forEach
is a powerful tool, it’s essential to be aware of its key points and limitations:
No New Array Returned: Unlike methods like
map
orfilter
,forEach
does not return a new array. Instead, it operates directly on the original array, potentially modifying its elements.Limitations:
forEach
lacks certain features found in other looping constructs, such as the ability to break or continue the loop. Additionally, it does not support reverse iteration out of the box, which can be a consideration in specific scenarios.Comparison with Other Array Methods: It’s crucial to compare
forEach
with other array methods likemap
,filter
, andreduce
to choose the most suitable tool for a given task. Each method has its unique strengths and use cases, and understanding them enables more informed decision-making.
Conclusion
In conclusion, the forEach
method offers a convenient and concise solution for iterating over arrays in JavaScript. Its simplicity, combined with its versatility, makes it a valuable asset for developers striving for clean and expressive code. By mastering forEach
, developers can streamline their array manipulation tasks and enhance the efficiency and readability of their JavaScript applications. Whether you’re iterating over elements to perform operations, transform data, or simply access array properties, forEach
provides an elegant and effective solution. So next time you encounter array iteration tasks in your JavaScript projects, consider harnessing the power of forEach
for a more productive coding experience.
Bonus: Advanced Usage Tips
For those seeking to optimize their usage of forEach
further, here are some advanced tips:
Arrow Functions: Leveraging arrow functions with
forEach
can enhance code readability and conciseness, especially for simple callback functions.const numbers = [1, 2, 3]; numbers.forEach(num => console.log(num * 2));
Customizing
this
Context: The optionalthisArg
parameter allows you to specify the value to be used asthis
within the callback function, providing flexibility in managing the execution context.Consider Alternatives: While
forEach
is powerful, there are scenarios where other array methods likemap
,filter
, andreduce
may offer more appropriate solutions. Understanding the strengths and weaknesses of each method empowers developers to choose the most suitable tool for their specific use case, optimizing code efficiency and maintainability.
Understanding Logical Operators in JavaScript
FAQs
A ForEach loop is a method used to iterate over elements of an array or array-like object in JavaScript. It executes a provided function once for each array element.
Unlike a traditional for loop, a ForEach loop does not require initialization, condition, or increment/decrement statements. Instead, it directly operates on each element of the array.
The syntax is as follows:
array.forEach(function(currentValue, index, array) {
// Your code here
});
The callback function is invoked once for each element in the array. It can take up to three arguments: currentValue (the current element being processed), index (the index of the current element), and array (the array being traversed).
You can define a function inside the ForEach loop that performs the desired action on each element. For example:
var array = [1, 2, 3, 4, 5];
array.forEach(function(element) {
console.log(element * 2); // Perform action (e.g., doubling each element)
});
No, the ForEach loop does not support breaking out prematurely. If you need to break out of a loop, consider using a traditional for loop or other loop constructs like for...of
or while
.
Yes, you can use control statements like return
to skip iterations based on certain conditions within the callback function. For example:
var array = [1, 2, 3, 4, 5];
array.forEach(function(element) {
if (element % 2 === 0) {
return; // Skip even numbers
}
console.log(element);
});
ForEach loops are generally slower than traditional for loops due to the overhead of calling the callback function for each element. In performance-critical scenarios, consider using other loop constructs for better optimization.
While ForEach is primarily designed for arrays, you can use it with array-like objects such as NodeList and arguments objects. However, it won’t work with objects or Map/Set data structures directly.
Yes, ForEach guarantees that elements are traversed in ascending order, starting from index 0 to the last index of the array. This order is consistent with the sequence of elements in the array.
Related Posts
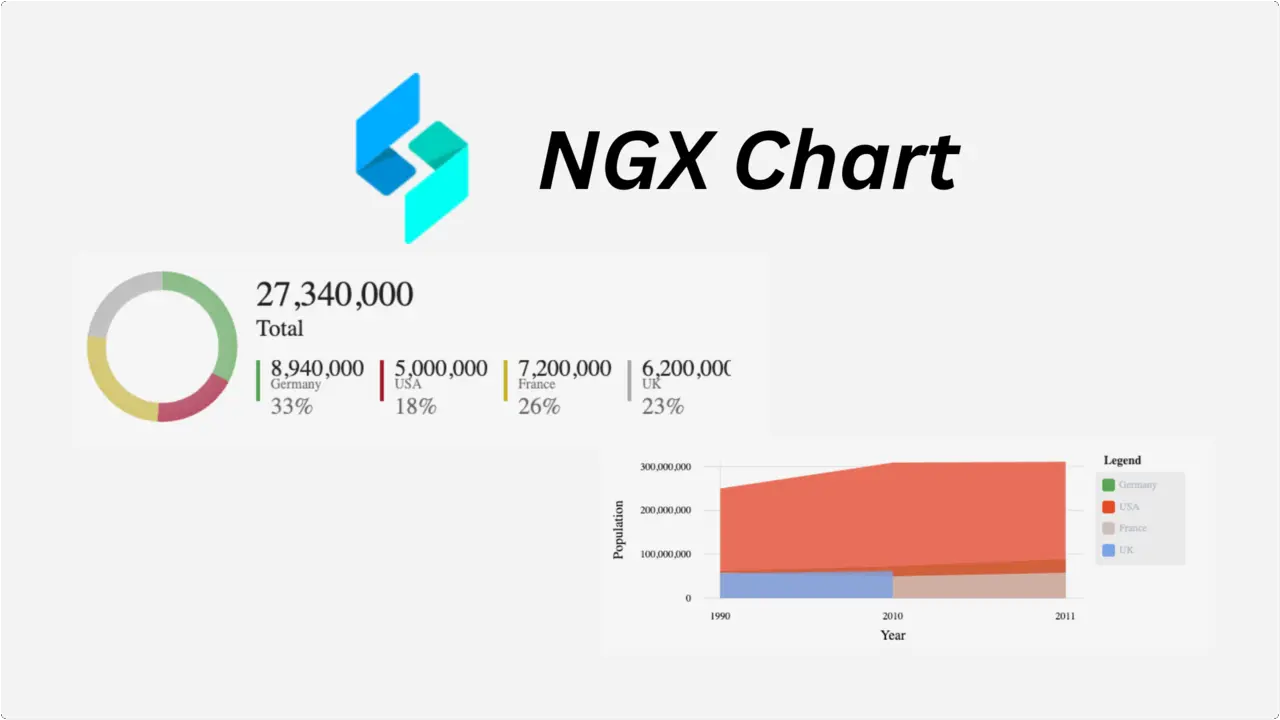
Start Using NGX Charts With Angular
Angular is a powerful front-end framework that enables developers to build robust and dynamic web applications. One essential aspect of modern web development is data visualization, and NGX Charts is a popular library that seamlessly integrates with Angular to create stunning charts and graphs.