JavaScript Debugging Techniques Beyond Console.Log
- With Code Example
- March 29, 2024
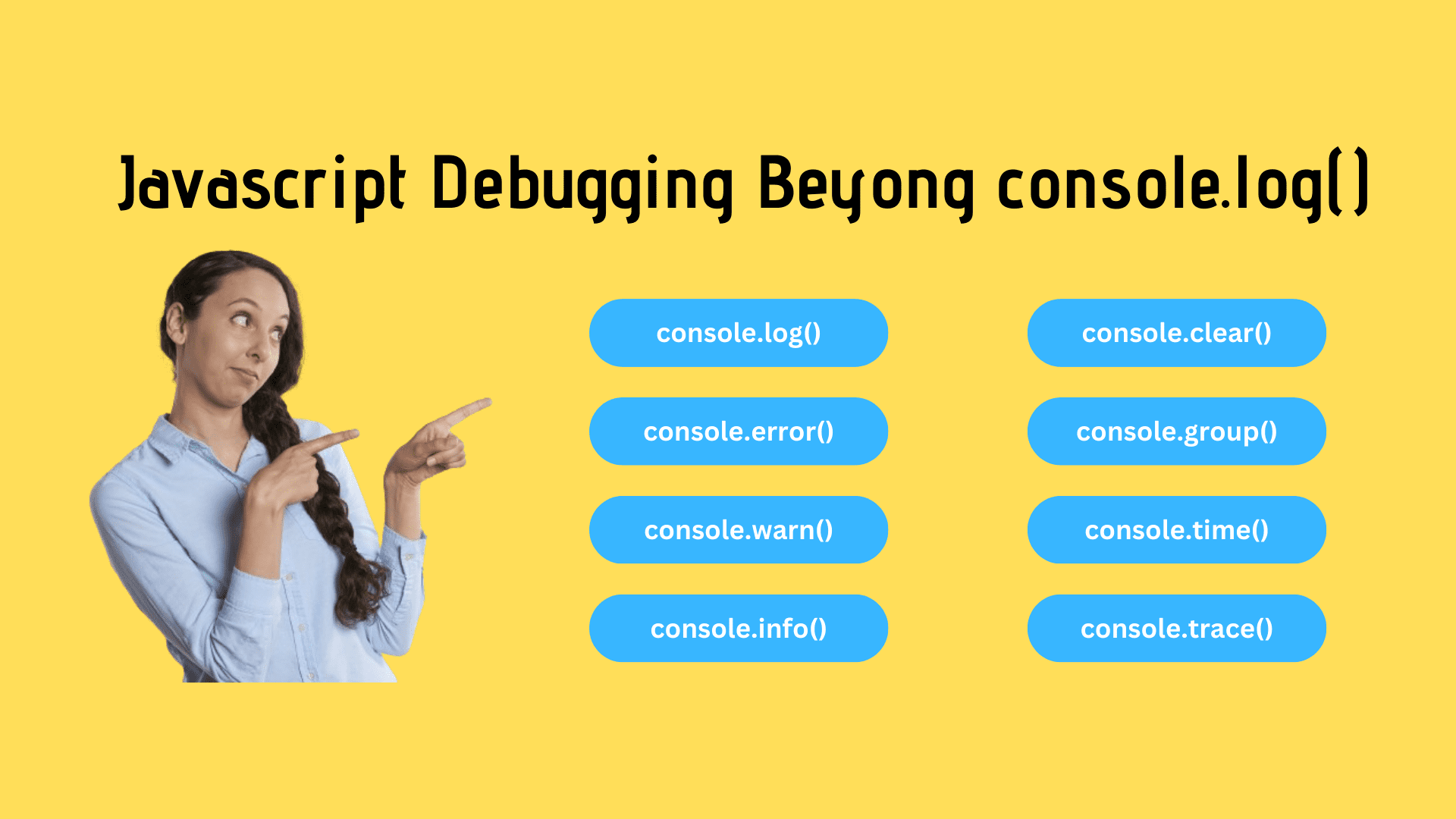
Hello Developers, I have decided to share some console
object methods that I am using to debug with JavaScript code in the developer tool. I have seen that most of the developers are using only console.log
to print all kinds of data, which is good but sometimes it is making our lives troubled. Javascript provides many types of console
object methods which you can you as per the data. So let’s get started to dive deep into the console
object methods in javascript
console.log()
We are already familiar with the console.log
object method, I think it is the most used in javascript debugging. It simply prints the data in the developer tool of your browser or the terminal in the case of node.js.
JavaScript console.log() Example
console.log("Hello, JavaScript")
Output
console.error()
The console.error()
is used to print the error message in the developer tool, see the example below
JavaScript console.error() Example
console.error("Hello, JavaScript")
Output
console.warn()
The console.warn()
is used to print the warning in the developer tool, see the example below
JavaScript console.warn() Example
console.warn("Hello, JavaScript")
Output
console.info()
The console.info()
is used to print the info in the developer tool, see the example below
JavaScript console.info() Example
console.info("Hello, JavaScript")
Output
console.clear()
The console.clear()
is used to clear the console, see the example below
JavaScript console.debug() Example
console.clear()
Output
console.table()
The console.table()
is used to show the data in tabular form, using console.table()
we can print the javascript or object in table form to rad it easily. Check the example below
JavaScript console.table() Example
console.table([{ name: 'John', age: 30 }, { name: 'Jane', age: 25 }]);
console.table({ name: 'Jane', age: 25 });
Output
console.group() and console.groupEnd()
The console.group()
and console.groupEnd()
is used to group the log messages together.
Javascript console.group() and console.groupEnd() example
console.group("Group");
console.log("Hello, JavaScript");
console.log("Hello, withcodeexample.com");
console.groupEnd();
Output
console.time()
This is also very useful when you want to calculate the time taken by a piece of code.
Javascript console.time() example
console.time("Timer");
for(var i = 0; i < 10; i++){
let j = i * i
}
console.timeEnd("Timer");
console.time("Timer");
for(var i = 0; i < 10000; i++){
let j = i * i
}
console.timeEnd("Timer");
Output
console.trace():
The console.trace()
outputs a stack trace to the console.
Javascript console.trace() example
function foo() {
console.trace();
}
foo();
Output
Ending Thoughts
So in this article on javascript debugging, I have provided almost all useful javascript console object methods which are useful and I am using them while working with javascript. I think it will be useful for you also, and it will help you print the log message neat and clean on the developer tools.
If you find this article useful please share it with your friends and colleagues to help them to write neat and clean javascript code. Thank you for reading, happy coding :)