JavaScript Reduce: A Comprehensive Guide
- With Code Example
- December 22, 2023
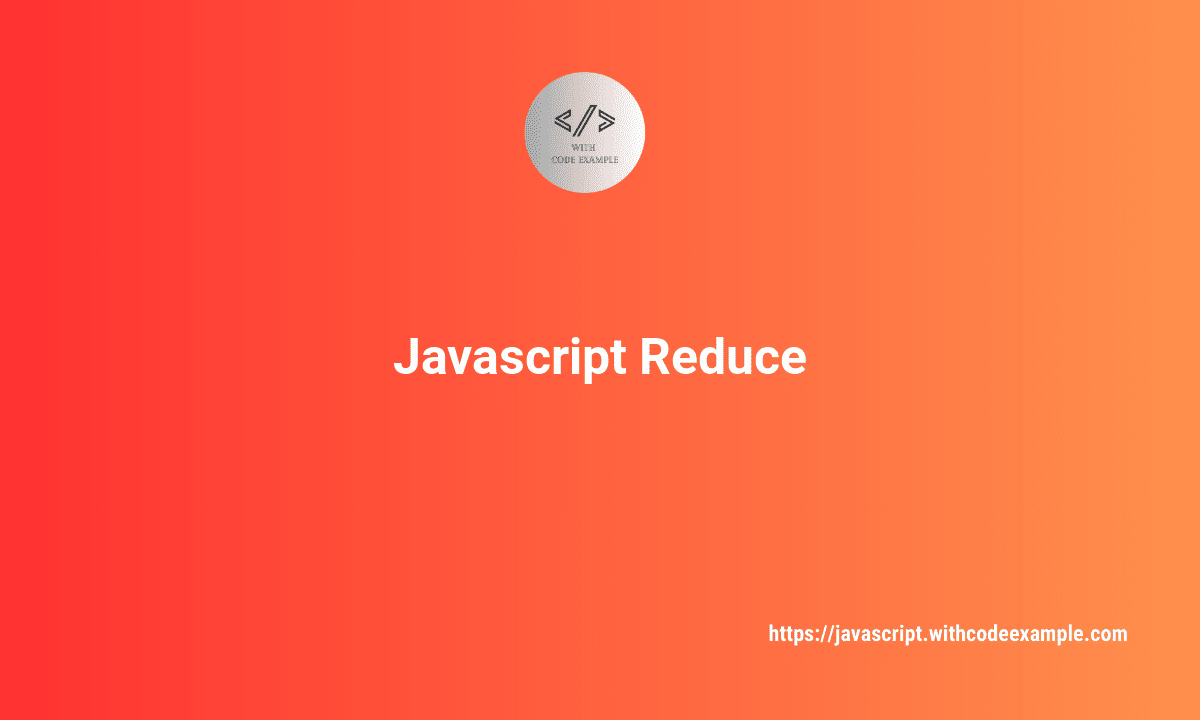
In the vast realm of JavaScript, the reduce
method stands as a powerful and versatile tool for array manipulation. Whether you are a seasoned developer or just starting your coding journey, understanding how to leverage reduce
can greatly enhance your programming prowess. In this comprehensive guide, we will delve into the intricacies of JavaScript’s reduce
method, providing you with insights, code examples, and practical applications.
What is reduce
?
At its core, the reduce
method is a higher-order function in JavaScript that iterates over each element of an array, accumulating a single result. This result can be anything from a primitive value like a number or string to a more complex data structure.
The Syntax of reduce
Let’s start by exploring the syntax of the reduce
method:
array.reduce(callback, initialValue);
Here, array
is the array on which you want to apply the reduce
method. The callback
function is executed for each element of the array, and the initialValue
is an optional parameter representing the initial value of the accumulator.
Now, let’s break down the components:
- array: The array you want to reduce.
- callback: A function that defines the logic for reducing. It takes four parameters: accumulator, currentValue, currentIndex, and the array itself.
- initialValue: An optional parameter that represents the initial value of the accumulator. If not provided, the first element of the array is used as the initial value.
Understanding the Callback Function
The heart of the reduce
method lies in the callback function. Let’s understand its parameters and their roles:
function callback(accumulator, currentValue, currentIndex, array) {
// Your logic here
return updatedAccumulator;
}
accumulator: This is the accumulated result of the callback’s previous executions. It starts with the
initialValue
or the first element of the array if no initial value is provided.currentValue: The current element being processed in the array.
currentIndex: The index of the current element being processed.
array: The array on which
reduce
is called.
The callback function executes for each element in the array, updating the accumulator based on your logic. It’s crucial to understand how to manipulate the accumulator to achieve the desired result.
Practical Examples
1. Summing Array Elements
Let’s start with a simple example: summing all the elements of an array.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(sum); // Output: 15
In this example, we initialize the accumulator to 0 and add each element of the array to it.
2. Flattening an Array
The reduce
method can also be used to flatten arrays, combining multiple arrays into a single one.
const nestedArrays = [[1, 2], [3, 4], [5, 6]];
const flattenedArray = nestedArrays.reduce((accumulator, currentArray) => {
return accumulator.concat(currentArray);
}, []);
console.log(flattenedArray); // Output: [1, 2, 3, 4, 5, 6]
Here, we use the concat
method to merge arrays, starting with an empty array as the initial value.
3. Grouping Array Objects by Property
Suppose you have an array of objects, and you want to group them based on a specific property.
const people = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 30 },
{ name: 'Charlie', age: 25 },
];
const groupedByAge = people.reduce((accumulator, person) => {
const key = person.age;
if (!accumulator[key]) {
accumulator[key] = [];
}
accumulator[key].push(person);
return accumulator;
}, {});
console.log(groupedByAge);
In this example, we group people by their age, creating an object where each key represents an age and the corresponding value is an array of people with that age.
Advantages of Using reduce
Conciseness and Readability: Using
reduce
often leads to more concise and readable code, especially when dealing with complex array manipulations.Flexibility: The flexibility of the
reduce
method allows you to implement a wide range of operations, from simple aggregations to more intricate transformations.Performance: In some scenarios, using
reduce
can be more performant than traditional methods likefor
loops.
Common Pitfalls and Best Practices
Pitfall 1: Forgetting the Initial Value
If your goal is to reduce an array to a single value and you forget to provide an initial value, unexpected results may occur.
const numbers = [1, 2, 3, 4, 5];
// Incorrect usage
const incorrectSum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
});
console.log(incorrectSum); // Output: 12345
In this example, the reduce
method treats the first element of the array as the initial value, resulting in concatenation instead of addition.
Best Practice 1: Always Provide an Initial Value
To avoid unexpected behavior, it’s a best practice to always provide an initial value, even if it’s 0
or an empty array.
const correctSum = numbers.reduce((accumulator, currentValue) => {
return accumulator + currentValue;
}, 0);
console.log(correctSum); // Output: 15
Pitfall 2: Mutating the Accumulator
Modifying the accumulator directly within the callback function can lead to unintended side effects.
const numbers = [1, 2, 3, 4, 5];
// Incorrect usage
const incorrectProduct = numbers.reduce((accumulator, currentValue) => {
accumulator *= currentValue;
return accumulator;
}, 1);
console.log(incorrectProduct); // Output: 120
In this case, the accumulator is unintentionally mutated, leading to incorrect results.
Best Practice 2: Avoid Mutation, Return a New Value
To maintain clarity and prevent unintended side effects, always return a new value instead of modifying the accumulator in place.
const correctProduct = numbers.reduce((accumulator, currentValue) => {
return accumulator * currentValue;
}, 1);
console.log(correctProduct); // Output: 120
Conclusion
In conclusion, mastering the reduce
method is a valuable skill for JavaScript developers. Its flexibility and power can streamline your code, making it more elegant and efficient. By understanding the syntax, callback function, and practical examples, you can harness the full potential of reduce
in your projects. Remember to follow best practices, avoid common pitfalls, and experiment with different use cases to become proficient in using reduce
effectively. Elevate your JavaScript coding experience by embracing the versatility of the reduce
method in your array manipulations.