Javascript Solid Principles
- With Code Example
- March 14, 2024
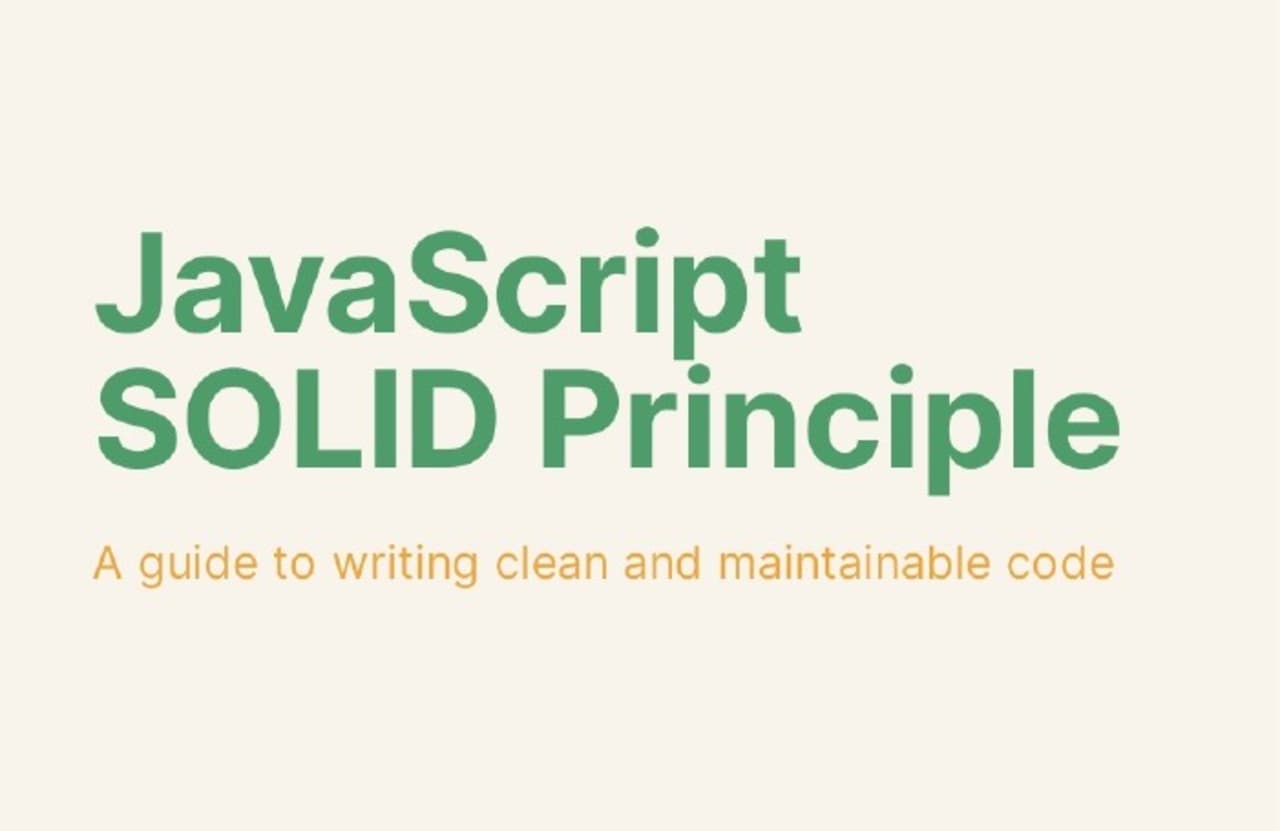
Series - Pdf
SOLID is an acronym that represents five fundamental principles of object-oriented design and programming. These principles aim to create maintainable, extensible, and testable software systems. The SOLID principles are:
Single Responsibility Principle (SRP): A class should have only one reason to change, meaning that it should have a single, well-defined responsibility. This principle promotes cohesion and helps to avoid code tangling.
Open/Closed Principle (OCP): Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means that you should be able to add new functionality without changing the existing code.
Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types. This means that if a class inherits from a base class, it should be able to be used in place of the base class without breaking the application.
Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use. This principle states that large monolithic interfaces should be split into smaller, more specific interfaces, so that clients only depend on the methods they actually use.
Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions. This principle promotes loose coupling between components and allows for easier code reuse and testability.
By adhering to the SOLID principles, developers can create code that is more maintainable, flexible, and easier to extend and refactor. These principles help to manage increasing complexity in software systems by promoting modular design, separation of concerns, and loose coupling between components.
It’s important to note that while SOLID principles are widely accepted guidelines, they should be applied judiciously and not treated as dogma. In some cases, violating one of the principles can be a pragmatic trade-off to meet specific project requirements or constraints.