JavaScript Array Length
- With Code Example
- August 10, 2023
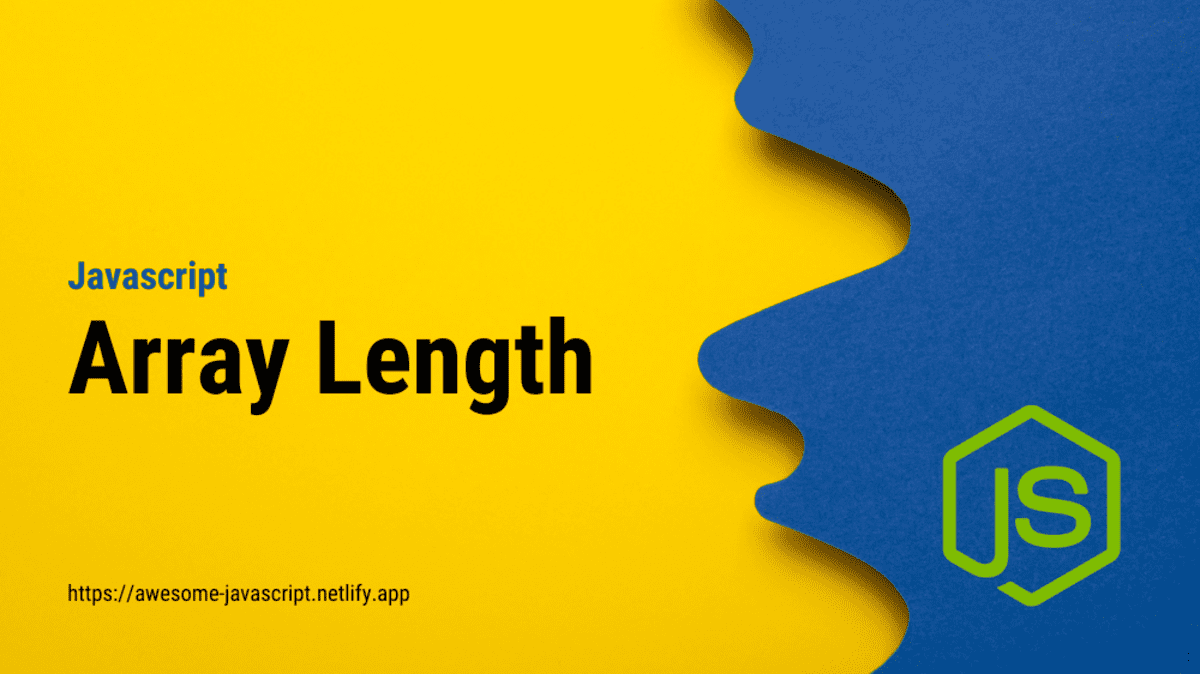
The JavaScript array is a versatile and fundamental data structure that lies at the heart of modern web development. While many developers are well-acquainted with the array’s power in storing multiple values, there’s a hidden gem within the realm of arrays that deserves its spotlight—the length
property. In this blog post, we’re embarking on a journey to uncover the magic of the length
property in JavaScript arrays. Buckle up, and let’s dive in!
1: The Basics of Array Length
Understanding the foundational concept of the length
property is essential. At its core, this property provides the count of elements contained within an array. By directly accessing the length
property, you gain valuable insights into the size of your array in real-time. Let’s illustrate this with a simple example:
const fruits = ['apple', 'banana', 'orange'];
const numberOfFruits = fruits.length;
console.log(`Number of fruits: ${numberOfFruits}`);
// Output: Number of fruits: 3
2: Dynamic Array Length Manipulation
The beauty of the length
property lies in its dynamic nature. Not only can you retrieve the array’s size, but you can also manipulate it to add or remove elements dynamically. This flexibility is a game-changer when working with evolving data. Observe how easily we can add and remove items:
const colors = ['red', 'blue', 'green'];
colors.push('yellow'); // Adding an element
console.log(colors.length); // Output: 4
colors.pop(); // Removing the last element
console.log(colors.length); // Output: 3
3: Array Length and Sparse Arrays
While arrays usually contain consecutive indices, JavaScript arrays can be “sparse.” In other words, they may have gaps in their indices. The length
property takes this into account and accurately reflects the highest index present, even if there are gaps. Consider this intriguing example:
const sparseArray = [];
sparseArray[5] = 'value';
console.log(sparseArray.length); // Output: 6
4: Performance Considerations
While the length
property is undoubtedly powerful, it’s wise to consider its impact on performance, especially when dealing with large arrays. When truncating an array using the length
property, keep in mind that it removes elements from the end. Therefore, it’s efficient for removing elements in LIFO (Last-In-First-Out) fashion. However, for more complex operations, alternative methods might offer better performance.
const numbers = [1, 2, 3, 4, 5];
numbers.length = 3; // Truncate array to 3 elements
console.log(numbers); // Output: [1, 2, 3]
5: Leveraging Array Length for Iteration
The length
property can be a valuable tool for iterating through arrays, enabling concise and efficient loops. By using the length
property to define the loop’s boundary, you ensure that every element is considered. Let’s witness this in action:
const names = ['Alice', 'Bob', 'Charlie', 'David'];
for (let i = 0; i < names.length; i++) {
console.log(`Hello, ${names[i]}!`);
}
Conclusion:
The length
property is more than just a simple numeric value; it’s a window into the dynamic world of JavaScript arrays. Its ability to provide size information, manipulate arrays, and optimize iteration makes it an indispensable asset in any developer’s toolkit. Armed with the insights from this exploration, you’re ready to harness the magic of the length
property and wield arrays with newfound confidence. Happy coding!