Node Authentication With Passport.Js
- With Code Example
- February 13, 2024
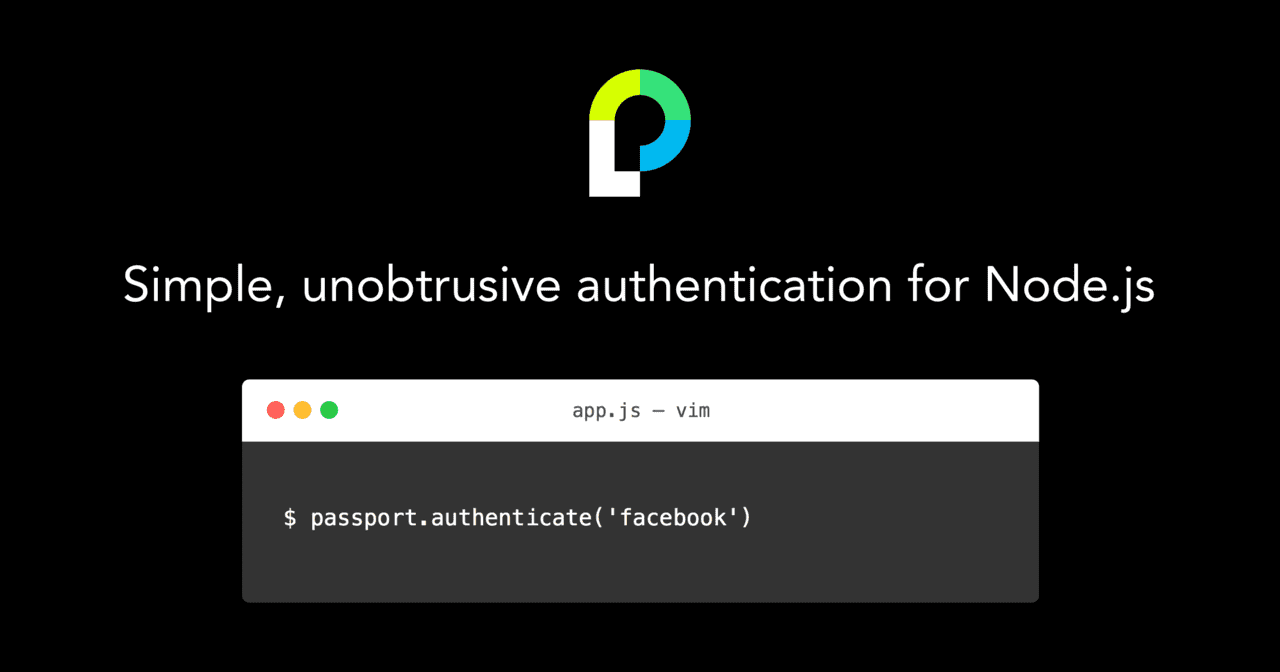
Passport.js is an authentication middleware for Node.js. It allows you to implement various authentication strategies in your Node.js application without having to build the logic from scratch.
Want to Learn Javascript ?
Some key features of Passport.js:
- Modular and flexible - Passport supports over 500 authentication strategies via plugins, allowing you to plug and play various auth mechanisms. Strategies support OAuth, OpenID, SAML, social logins and more.
- Unobtrusive - Passport does not impose itself on your application. It is designed to be a companion library that sits alongside Express or any other Node web framework.
- Supports sessions - Passport maintains user state via sessions, and will establish sessions as part of the login process. Common session stores like Redis are supported.
- Simple to use - Setting up Passport involves configuring the strategies you want, then writing middleware to initiate authentication and handle callbacks. The API is straightforward.
- Cross-platform - Passport is database agnostic and works across any platform that Node.js runs on.
To use Passport.js, you first install it via npm. You would then configure the specific authentication strategies your app needs, like Facebook Auth or Google Auth. When a user tries to access a protected route, they are redirected to the login page for that strategy. Upon successful auth, they are returned to your app where they can access protected resources. Passport persists the user ID in the session to maintain authentication state.
Benefits of using Passport.js:
- Saves development time: By using pre-built strategies and a well-structured codebase, you can focus on your application’s core functionality.
- Improves security: Passport.js follows best practices for secure authentication, helping you avoid common security vulnerabilities.
- Enhances user experience: Provides a smooth and user-friendly authentication process for your users.
Installing and Using Passport.js with Example
{.gif}
Here’s a basic example of installing and using Passport.js with local username/password authentication:
1. Installation:
First, install Passport.js and the passport-local
strategy using npm or yarn:
Bash
npm install passport passport-local
2. Server Setup:
Assuming you’re using Express.js, set up your server and require the necessary modules:
const express = require('express');
const passport = require('passport');
const LocalStrategy = require('passport-local').Strategy;
const app = express();
// ... your other app setup (middleware, routes, etc.)
3. Configure Passport:
1. Initialize Passport:
app.use(passport.initialize());
2. Define the Authentication Strategy:
Create a LocalStrategy instance, providing a callback function to verify user credentials (usually against a database):
passport.use(new LocalStrategy(
(username, password, done) => {
// Replace this with your logic to check username and password in your database
if (username === 'admin' && password === 'password') {
return done(null, { username: username }); // Successful authentication
} else {
return done(null, false); // Failed authentication
}
}
));
**Note:** This is a simplified example for demonstration purposes. In real applications, you should follow secure password storage practices like hashing and salting.
3. Serialize and Deserialize User:
Tell Passport how to store and retrieve user data in and out of sessions:
passport.serializeUser((user, done) => {
done(null, user.id); // Store user ID in session
});
passport.deserializeUser((id, done) => {
// Replace this with your logic to fetch user data from your database based on ID
done(null, { username: 'admin' }); // Retrieved user
});
4. Implement Login Route:
Create a route for handling login requests and using Passport for authentication:
app.post('/login', passport.authenticate('local', {
failureRedirect: '/login-error', // Redirect on failure
}), (req, res) => {
// Successful authentication, redirect to protected route or home page
res.redirect('/');
});
5. Protect Routes:
Use middleware to protect routes that require authentication:
app.get('/protected', passport.authenticate('local'), (req, res) => {
// User is authenticated, access protected resources
res.send('Welcome to the protected area!');
});
6. Run the Server:
Start your Node.js server to test the implemented authentication flow.
Remember: This is a basic example and needs adjustments based on your specific database and security requirements.
Additional Resources:
- Passport.js Documentation: https://www.passportjs.org/docs/
- Express.js Tutorial: https://expressjs.com/en/starter/hello-world.html
By following these steps and exploring the resources provided, you should be able to get started with implementing user authentication using Passport.js in your Node.js applications.
Conclusion
Passport.js is a powerful and versatile authentication middleware for Node.js applications. Its modular design, extensive list of supported strategies, and focus on security make it an excellent choice for developers looking to streamline user authentication and authorization. Whether you need basic local username/password login or integration with social media platforms and OAuth providers, Passport.js has the tools and flexibility to meet your needs.
By leveraging Passport.js, you can save development time, improve security, and enhance the user experience of your application. With its active community and comprehensive documentation, getting started with Passport.js is easy, and its wide range of features ensures it can grow with your application’s complexity.
If you’re building a Node.js application that requires user authentication, Passport.js is definitely worth considering. It’s a robust and well-regarded solution that can help you implement secure and user-friendly authentication in your projects.
FAQs
Passport.js is a popular Node.js middleware for authentication. It simplifies the process of adding authentication to your application by providing a unified API for different authentication strategies.
There are several benefits to using Passport.js, including:
Simplicity: Passport.js provides a unified API for different authentication strategies, making it easy to add authentication to your application.
Security: Passport.js helps improve the security of your application by providing a number of features, such as session management and CSRF protection.
Flexibility: Passport.js supports a wide range of authentication strategies, so you can choose the one that best suits your needs.
You can install Passport.js using npm or yarn:
npm install passport
Using Passport.js involves several steps:
- Install Passport.js and any required authentication strategies.
- Configure Passport.js with your authentication strategy.
- Use Passport.js middleware in your routes to protect them.