Unlocking the Power of React Hooks: A Comprehensive Guide
- With Code Example
- August 14, 2023
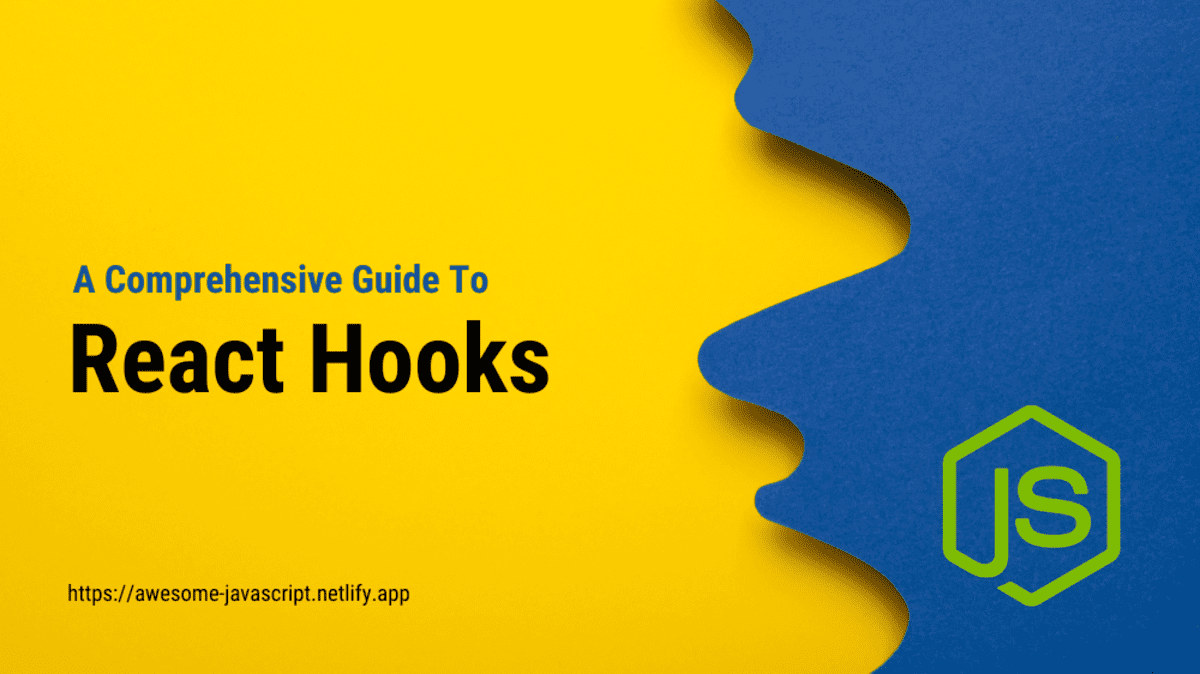
Series - React Hooks
React Hooks are a set of functions that allow you to add state and other React features to functional components. They were introduced in React 16.8 as a way to use stateful logic in functional components without the need for class components. Hooks provide a more concise and intuitive way to manage state, effects, context, and more. Let’s dive deeper into some of the most commonly used React Hooks:
1. useState:
useState
is used to manage state within a functional component. It returns a state variable and a function to update that variable. This hook allows you to add state to functional components, enabling them to maintain dynamic data.
useState Example:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
Learn useState in details with example
2. useEffect:
useEffect
is used for handling side effects in functional components. It replaces lifecycle methods like componentDidMount
, componentDidUpdate
, and componentWillUnmount
. It’s used to perform tasks like data fetching, DOM manipulation, and more.
useEffect Example:
import React, { useState, useEffect } from 'react';
const DataFetchingComponent = () => {
const [data, setData] = useState([]);
useEffect(() => {
// Fetch data from an API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => setData(data));
}, []); // Empty array means this effect runs only once (on mount)
return (
<div>
{data.map(item => (
<p key={item.id}>{item.name}</p>
))}
</div>
);
};
Learn useEffect in details with example
3. useContext:
useContext
allows you to access the context of a parent component within a child component. Context is a way to share data between components without passing props manually at every level.
useContext Example:
import React, { useContext } from 'react';
const ThemeContext = React.createContext();
const ThemedButton = () => {
const theme = useContext(ThemeContext);
return (
<button style={{ backgroundColor: theme.background, color: theme.foreground }}>
Themed Button
</button>
);
};
const App = () => (
<ThemeContext.Provider value={{ background: 'lightblue', foreground: 'darkblue' }}>
<ThemedButton />
</ThemeContext.Provider>
);
Learn useContext in details with example
4. useReducer:
useReducer
is an alternative to useState
when dealing with complex state logic. It’s particularly useful for managing state transitions in a predictable manner, similar to how Redux manages state.
useReducer Example:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
const Counter = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
Learn useReducer in details with example
React Hooks provide a modern and powerful way to work with state and effects in functional components, making them more readable and easier to maintain. By understanding and using these hooks effectively, you can create robust and efficient React applications.