Start Using NGX Charts With Angular
- With Code Example
- March 1, 2024
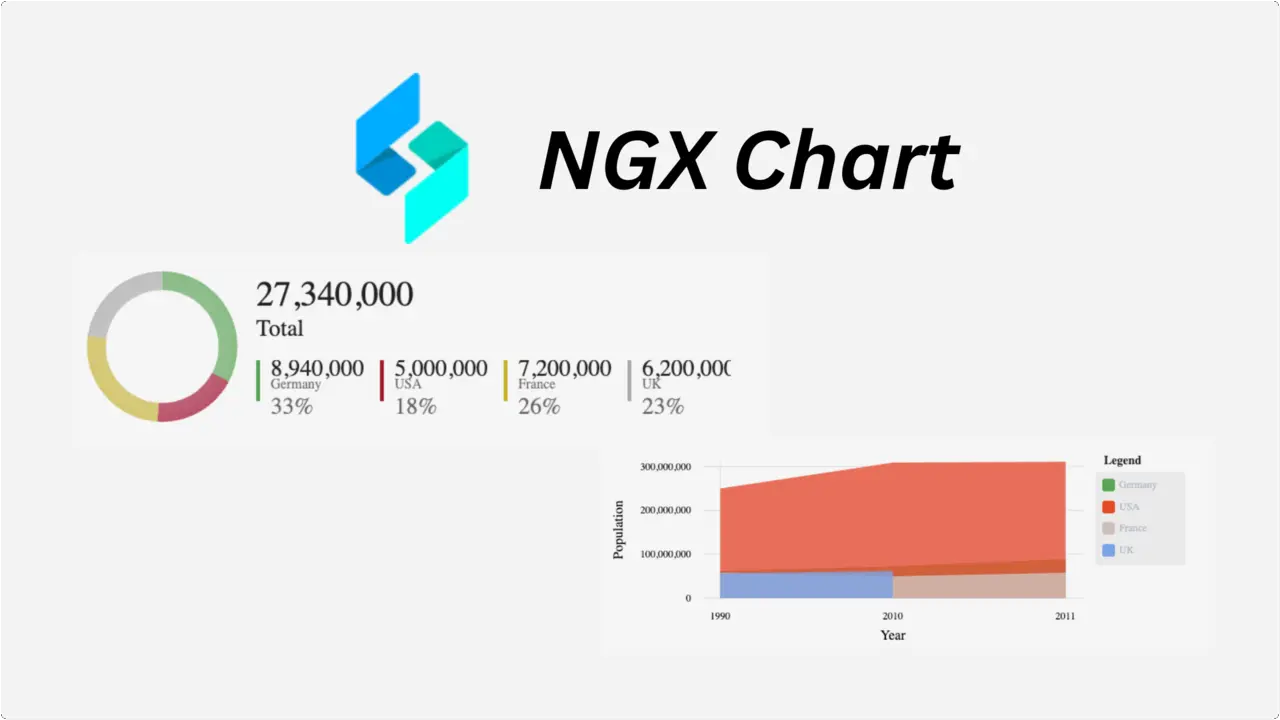
Series - NGX Charts
Angular is a powerful front-end framework that enables developers to build robust and dynamic web applications. One essential aspect of modern web development is data visualization, and NGX Charts is a popular library that seamlessly integrates with Angular to create stunning charts and graphs. In this comprehensive guide, we will delve into the fundamentals of NGX Charts and explore how to incorporate it into your Angular projects effectively.
Table of Contents
Note
This comprehensive guide has covered the essentials of integrating NGX Charts into your Angular projects, from installation and configuration to advanced customization and integration. By following the step-by-step instructions and exploring the provided examples, you should now have a solid understanding of how to leverage NGX Charts to create beautiful and interactive charts and graphs in your Angular applications.
Features
Initially, our aim was to establish a sturdy, foundational framework for constructing charts at a low level. This framework is designed to accommodate various chart types, with exceptions being:
- Chart Types
- Customization
Chart Types
- Horizontal & Vertical Bar Charts (Standard, Grouped, Stacked, Normalized)
- Line
- Area (Standard, Stacked, Normalized)
- Pie (Explodable, Grid, Custom legends)
- Donut
- Gauge
- Linear Gauge
- Force Directed Graph (deprecated - please use instead)
- Heatmap
- Treemap
- Number Cards
- Bubble/Scatter
- Vertical Box Chart
Customization
- Autoscaling
- Timeline Filtering
- Line Interpolation
- Configurable Axis Labels
- Legends (Labels & Gradient)
- Advanced Label Positioning
- Real-time data support
- Advanced Tooltips
- Responsive Layout
- Data point Event Handlers
- Works with ngUpgrade
- Enabling/Disabling animations
Getting Started with NGX Charts
To begin harnessing the capabilities of NGX Charts in your Angular application, the first step is to install the library. You can easily install NGX Charts using npm, a package manager for Node.js modules. Open your terminal and navigate to your Angular project directory. Then, execute the following command:
npm install ngx-charts --save
This command will download and install the NGX Charts library along with its dependencies, making it available for use in your Angular application.
NGX Bar Chart example
- app.module.ts
- app.component.html
- app.component.ts
Once NGX Charts is installed, you need to import the necessary modules into your Angular application to utilize its components effectively. In your Angular module file (e.g., app.module.ts
), import the NgxChartsModule
from ngx-charts
as shown below:
import { NgxChartsModule } from '@swimlane/ngx-charts';
@NgModule({
declarations: [
// Your components here
],
imports: [
// Other modules
NgxChartsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
By importing NgxChartsModule
, you ensure that NGX Charts components and directives are available throughout your Angular application.
With NGX Charts imported into your Angular application, you can now proceed to create your first chart. Let’s start by adding a simple bar chart to visualize some sample data. In your component template file (e.g., app.component.html
), include the following NGX Charts component:
<ngx-charts-bar-vertical
[view]="[500,300]"
[results]="barChartData">
</ngx-charts-bar-vertical>
In your component class (e.g., app.component.ts
), define the sample data for the bar chart:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
barChartData = [
{ name: 'A', value: 10 },
{ name: 'B', value: 20 },
{ name: 'C', value: 15 }
];
}
By binding the results
property of the ngx-charts-bar-vertical
component to the barChartData
array in your component class, you provide the data source for the bar chart.
Customizing NGX Charts Components
One of the key advantages of NGX Charts is its flexibility in customization. You can easily customize the appearance and behavior of NGX Charts components to suit your specific requirements. Let’s explore some common customization options for NGX Charts components.
1. Styling Charts: NGX Charts provides various styling options to customize the appearance of charts. You can modify colors, fonts, and other visual properties using CSS. For example, to change the color palette of a bar chart, you can override the default styles:
::ng-deep .bar {
fill: #007bff; /* Change bar color to blue */
}
2. Configuring Axes: NGX Charts allows you to configure axes to display labels, ticks, and gridlines according to your preferences. You can customize axis properties such as scale type, tick formatting, and orientation.
<ngx-charts-bar-vertical
[view]="[500,300]"
[results]="barChartData"
[xAxis]="true"
[yAxis]="true"
[showXAxisLabel]="true"
[showYAxisLabel]="true"
xAxisLabel="X Axis Label"
yAxisLabel="Y Axis Label">
</ngx-charts-bar-vertical>
3. Handling Events: NGX Charts components emit events that allow you to handle user interactions such as clicks, hovers, and selections. You can listen to these events in your component class and perform custom actions based on user interactions.
<ngx-charts-bar-vertical
[view]="[500,300]"
[results]="barChartData"
(select)="onChartSelect($event)">
</ngx-charts-bar-vertical>
onChartSelect(event: any): void {
console.log('Chart selected:', event);
}
By leveraging these customization options, you can tailor NGX Charts components to meet the specific requirements of your Angular application.
Advanced Usage and Integration
Beyond basic chart creation and customization, NGX Charts offers advanced features and seamless integration with other Angular libraries and frameworks. Let’s explore some advanced usage scenarios and integration possibilities.
1. Dynamic Data Binding: NGX Charts supports dynamic data binding, allowing you to update chart data in real-time based on user input or external data sources. You can use Angular’s data binding features such as property binding and event binding to achieve dynamic updates.
2. Integration with Angular Material: NGX Charts can be seamlessly integrated with Angular Material, a UI component library for Angular applications. By combining NGX Charts with Angular Material components, you can create cohesive and visually appealing user interfaces.
3. Server-Side Rendering: NGX Charts is compatible with server-side rendering (SSR) techniques, enabling you to render charts on the server side for improved performance and SEO. You can use frameworks like Angular Universal to implement SSR with NGX Charts.
Conclusion
In conclusion, NGX Charts is a powerful and versatile library for data visualization in Angular applications. By following the steps outlined in this guide, you can easily integrate NGX Charts into your Angular projects and create interactive and visually stunning charts and graphs. With its extensive customization options and seamless integration capabilities, NGX Charts empowers developers to deliver compelling data-driven experiences to users.