Exploring the useContext Hook in React
- With Code Example
- August 17, 2023
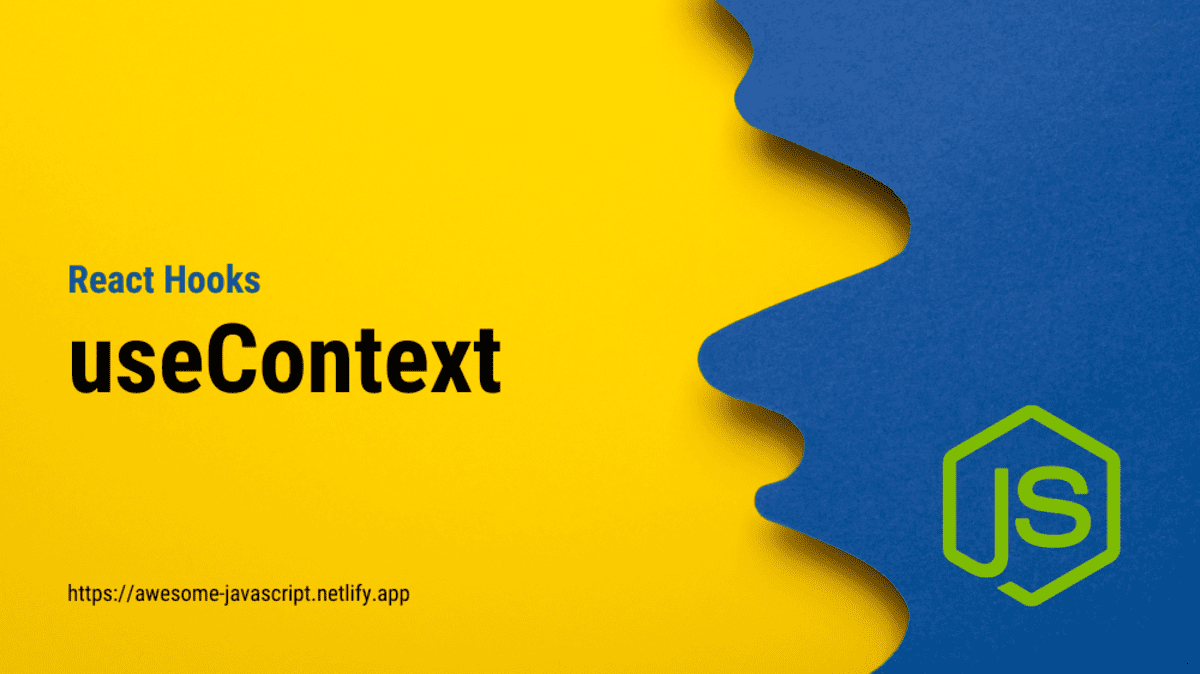
Series - React Hooks
The useContext
hook in React allows you to access the context of a parent component within a child component, without the need for prop drilling. This is particularly useful for sharing data or state between components that are not directly related in the component tree. Let’s explore a couple of examples of how to use the useContext
hook.
Theme Context
Suppose you want to create a themed user interface where components can access the theme colors defined at a higher level. Here’s how you can achieve that using useContext
:
1. Create a Context:
import React, { createContext } from 'react';
const ThemeContext = createContext();
export default ThemeContext;
2. Provide the Context in a Parent Component:
import React from 'react';
import ThemeContext from './ThemeContext';
const App = () => {
const theme = {
background: 'lightblue',
foreground: 'darkblue'
};
return (
<ThemeContext.Provider value={theme}>
{/* Your component hierarchy */}
</ThemeContext.Provider>
);
};
3. Use the Context in Child Components:
import React, { useContext } from 'react';
import ThemeContext from './ThemeContext';
const ThemedButton = () => {
const theme = useContext(ThemeContext);
return (
<button style={{ backgroundColor: theme.background, color: theme.foreground }}>
Themed Button
</button>
);
};
User Authentication Context
Imagine you’re building an authentication system, and you want to share the user’s authentication status and related functions across various components. Here’s how you can achieve this using useContext
:
1. Create a Context:
import React, { createContext, useContext, useState } from 'react';
const AuthContext = createContext();
const AuthProvider = ({ children }) => {
const [isLoggedIn, setIsLoggedIn] = useState(false);
const login = () => {
setIsLoggedIn(true);
};
const logout = () => {
setIsLoggedIn(false);
};
return (
<AuthContext.Provider value={{ isLoggedIn, login, logout }}>
{children}
</AuthContext.Provider>
);
};
export { AuthContext, AuthProvider };
2. Provide the Context in a Parent Component:
import React from 'react';
import { AuthProvider } from './AuthContext';
const App = () => {
return (
<AuthProvider>
{/* Your component hierarchy */}
</AuthProvider>
);
};
3. Use the Context in Child Components:
import React, { useContext } from 'react';
import { AuthContext } from './AuthContext';
const Profile = () => {
const { isLoggedIn, login, logout } = useContext(AuthContext);
return (
<div>
{isLoggedIn ? (
<div>
<p>Welcome, User!</p>
<button onClick={logout}>Logout</button>
</div>
) : (
<button onClick={login}>Login</button>
)}
</div>
);
};
These examples demonstrate how you can use the useContext
hook to share data and functions across components efficiently, without passing props through every level of the component tree. This helps in keeping your codebase clean and maintainable.
Related Posts
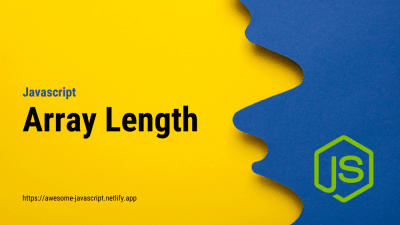
JavaScript Array Length
The JavaScript array is a versatile and fundamental data structure that lies at the heart of modern web development. While many developers are well-acquainted with the array’s power in storing multiple values, there’s a hidden gem within the realm of arrays that deserves its spotlight—the length property.
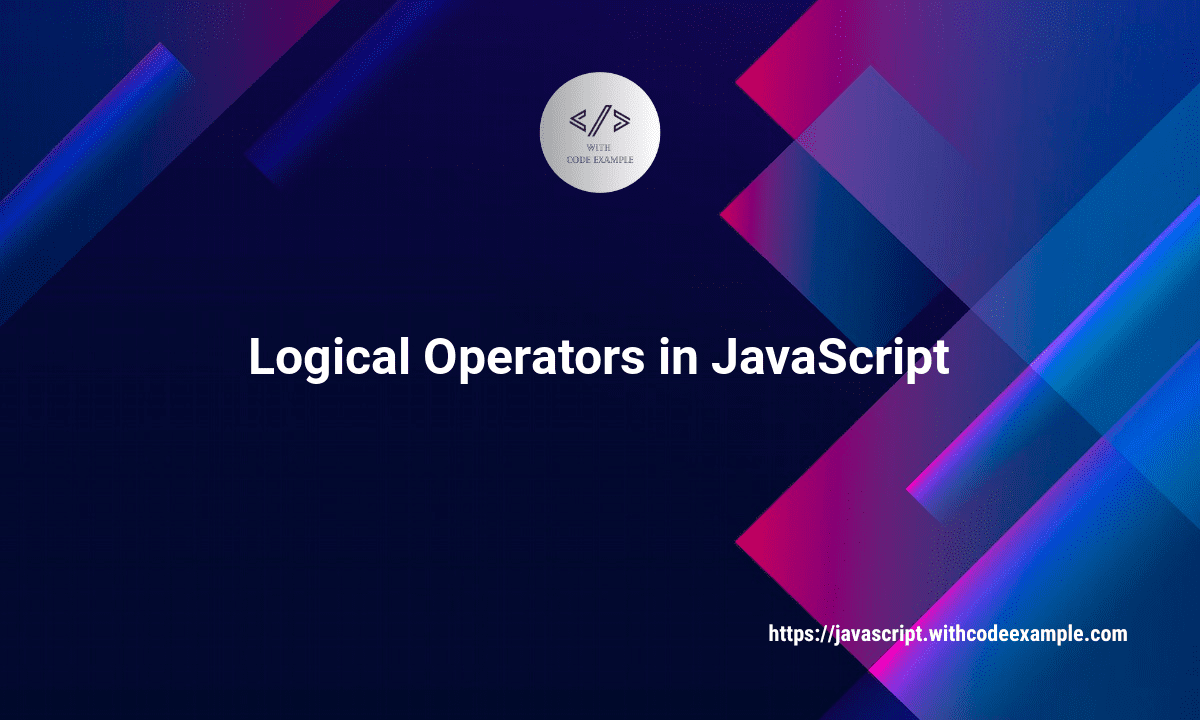
Understanding Logical Operators in JavaScript
When it comes to building robust and dynamic web applications, mastering the art of conditional logic is essential. JavaScript, as one of the primary languages of web development, offers a suite of logical operators that enable developers to create sophisticated decision-making processes.